What is a Python defaultdict?
A defaultdict is a subclass of the dictionary class that returns a default value for all non-existing keys.
It is useful when you want to avoid the KeyError exception that is raised when you try to access a non-existing key in a dictionary.
The default value for a defaultdict can be any object, including another dictionary, a list, or a set.
Here is an example of how to use a defaultdict:
pythonfrom collections import defaultdict# Create a defaultdict with a default value of 0d = defaultdict(int)# Add some key-value pairs to the dictionaryd['a'] = 1d['b'] = 2# Get the value for a key that does not existprint(d['c']) # Output: 0
In this example, we create a defaultdict with a default value of 0. Then, we add some key-value pairs to the dictionary. Finally, we get the value for a key that does not exist. The default value of 0 is returned.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors.
Python defaultdict
A defaultdict is a subclass of the dictionary class that returns a default value for all non-existing keys. This can be useful in a variety of situations, such as when you want to avoid the KeyError exception that is raised when you try to access a non-existing key in a dictionary.
- Default value: The default value for a defaultdict can be any object, including another dictionary, a list, or a set.
- Creation: Defaultdicts are created using the defaultdict() function from the collections module.
- Access: You can access the value for a key in a defaultdict using the [] operator. If the key does not exist, the default value will be returned.
- Setting: You can set the value for a key in a defaultdict using the [] operator. If the key does not exist, it will be created automatically.
- Deletion: You can delete a key from a defaultdict using the del operator.
- Iteration: You can iterate over the keys and values in a defaultdict using the keys() and values() methods.
- Comprehension: You can use defaultdict comprehensions to create new defaultdicts.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Default value
This feature of defaultdict makes it a powerful tool for working with data that may have missing values. By specifying a default value, you can avoid the KeyError exception that is raised when you try to access a non-existing key in a dictionary.
- Using a default value as another dictionary:
You can use a defaultdict with a default value of another dictionary to create a nested dictionary structure. This can be useful for organizing data that has a hierarchical structure. - Using a default value as a list:
You can use a defaultdict with a default value of a list to create a dictionary that automatically creates a list for missing keys. This can be useful for collecting data from multiple sources. - Using a default value as a set:
You can use a defaultdict with a default value of a set to create a dictionary that automatically creates a set for missing keys. This can be useful for finding unique values in a dataset.
Overall, the ability to specify a default value for a defaultdict makes it a versatile tool for working with data in Python.
Creation
Defaultdicts are created using the defaultdict() function from the collections module. This function takes two arguments: the default factory and the optional keyword arguments that are passed to the default factory.
- Default factory:The default factory is a function or class that is called to create the default value for a non-existing key. The default factory can be any object, including another dictionary, a list, or a set.
- Keyword arguments:The keyword arguments are passed to the default factory when it is called to create the default value for a non-existing key.
Here is an example of how to create a defaultdict with a default value of 0:
pythonfrom collections import defaultdictd = defaultdict(int)
This defaultdict will automatically create a value of 0 for any non-existing key.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Access
The ability to access the value for a key in a defaultdict using the [] operator is a fundamental feature of defaultdicts. It allows you to easily retrieve the value for a key, even if the key does not exist. This can be very useful in situations where you need to work with dictionaries that may contain missing keys.
For example, consider a defaultdict with a default value of 0. You can use this defaultdict to keep track of the number of times a particular word appears in a document. To do this, you would simply access the value for the word using the [] operator. If the word does not exist in the defaultdict, the default value of 0 will be returned. You can then increment the value to keep track of the number of times the word appears.
The ability to access the value for a key in a defaultdict using the [] operator is a powerful feature that makes defaultdicts a versatile tool for working with data in Python.
Here are some additional examples of how you can use the [] operator to access the value for a key in a defaultdict:
- To get the value for a key, you can use the following syntax:```pythond[key]```
- To set the value for a key, you can use the following syntax:```pythond[key] = value```
- To delete a key, you can use the following syntax:```pythondel d[key]```
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Setting
The ability to set the value for a key in a defaultdict using the [] operator is a fundamental feature of defaultdicts. It allows you to easily add new key-value pairs to a defaultdict, even if the key does not exist. This can be very useful in situations where you need to work with dictionaries that may contain missing keys.
- Creating new key-value pairs:
You can use the [] operator to create new key-value pairs in a defaultdict. If the key does not exist, the default value will be returned. You can then set the value for the key to the desired value. - Updating existing key-value pairs:
You can also use the [] operator to update the value for an existing key in a defaultdict. Simply set the value for the key to the new value. - Deleting key-value pairs:
You can delete a key-value pair from a defaultdict using the del operator. Simply delete the key from the defaultdict.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Deletion
The del operator can be used to delete a key from a defaultdict. This can be useful in situations where you need to remove a key-value pair from a defaultdict.
- Removing a key-value pair:
You can use the del operator to remove a key-value pair from a defaultdict. Simply delete the key from the defaultdict. - Deleting a key that does not exist:
If you try to delete a key that does not exist in a defaultdict, the KeyError exception will be raised.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Iteration
Iteration is an important operation for working with dictionaries. Defaultdicts support iteration over the keys and values in the dictionary using the keys() and values() methods, respectively.
- Iterating over keys:
The keys() method returns an iterator over the keys in the defaultdict. This can be useful for looping over the keys in the dictionary to perform some operation, such as printing the keys or checking if a particular key exists. - Iterating over values:
The values() method returns an iterator over the values in the defaultdict. This can be useful for looping over the values in the dictionary to perform some operation, such as printing the values or summing the values.
Defaultdicts are a powerful tool that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Comprehension
Defaultdict comprehensions are a concise way to create new defaultdicts. They are similar to list comprehensions and dictionary comprehensions, but they create defaultdicts instead. The syntax for a defaultdict comprehension is as follows:
pythond = {key: defaultdict(factory) for key in keys}
Where:
- d is the new defaultdict.
- key is the key for the new defaultdict.
- factory is the default factory for the new defaultdict.
For example, the following code creates a defaultdict that maps each letter in the alphabet to a list:
pythond = {letter: defaultdict(list) for letter in string.ascii_lowercase}
This defaultdict can be used to store data in a structured way. For example, you could use it to store the anagrams of each letter in the alphabet. To do this, you would simply add the anagrams of each letter to the corresponding list in the defaultdict.
Defaultdict comprehensions are a powerful tool that can be used to create complex data structures in a concise and efficient way. They are particularly useful for creating defaultdicts that have a nested structure.
FAQs about Python defaultdict
Defaultdicts are a powerful data structure in Python that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Question 1: What is a defaultdict?
A defaultdict is a subclass of the dictionary class that returns a default value for all non-existing keys.
Question 2: How do I create a defaultdict?
Defaultdicts are created using the defaultdict() function from the collections module.
Question 3: How do I access the value for a key in a defaultdict?
You can access the value for a key in a defaultdict using the [] operator. If the key does not exist, the default value will be returned.
Question 4: How do I set the value for a key in a defaultdict?
You can set the value for a key in a defaultdict using the [] operator. If the key does not exist, it will be created automatically.
Question 5: How do I delete a key from a defaultdict?
You can delete a key from a defaultdict using the del operator.
Question 6: How do I iterate over the keys and values in a defaultdict?
You can iterate over the keys and values in a defaultdict using the keys() and values() methods, respectively.
Summary: Defaultdicts are a powerful data structure in Python that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
Next steps: To learn more about defaultdicts, you can refer to the Python documentation or search for tutorials and examples online.
Conclusion
In this article, we explored the defaultdict class from the collections module in Python. We discussed how to create defaultdicts, access and set values, delete keys, iterate over keys and values, and use defaultdict comprehensions.
Defaultdicts are a powerful data structure that can be used to simplify your code and avoid errors. They are particularly useful in situations where you need to work with dictionaries that may contain missing keys.
We encourage you to experiment with defaultdicts in your own code to see how they can help you write more concise and efficient code.
What Constantinople Was Historically Called: A Journey Through Time
The Ultimate Guide To Case Studies Of Single-Keyword SEO
Learn How To Say Twenty In English: A Comprehensive Guide

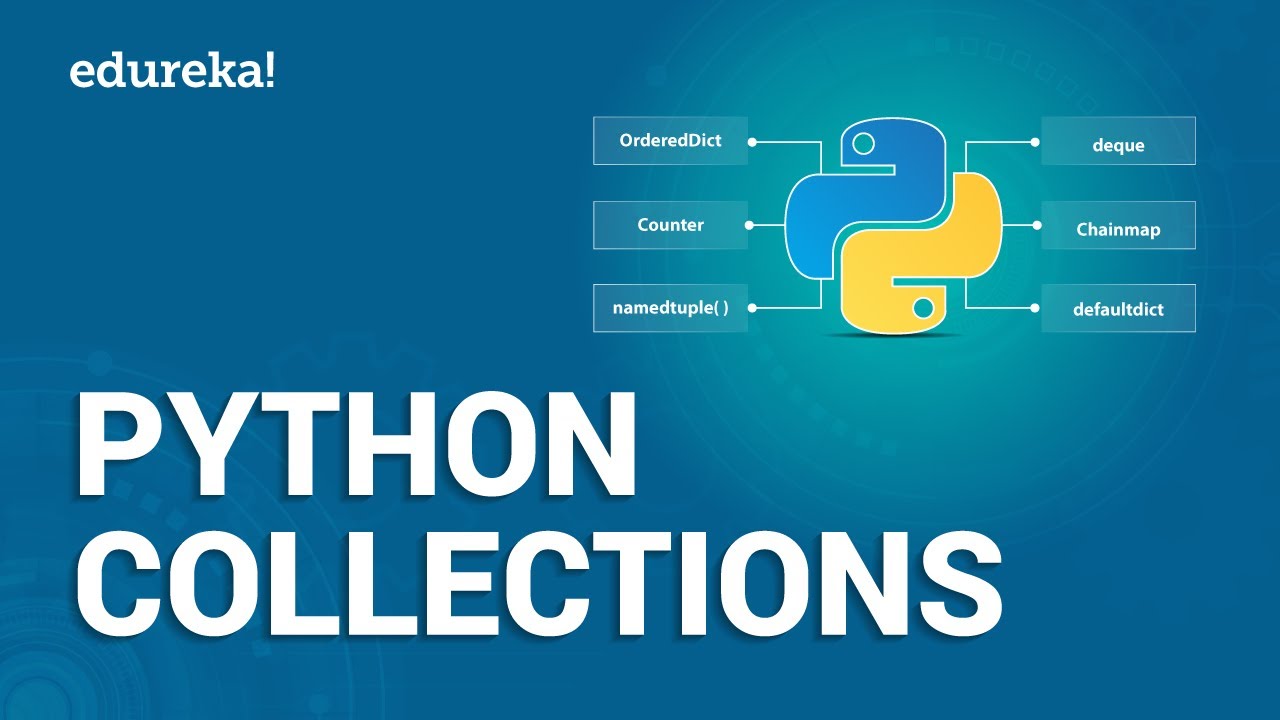