What is the return type of a switch to method in C# for window handles?
The return type of a switch to method in C# for window handles is `IntPtr`. This is because window handles are represented as `IntPtr` values in C#.
Here is an example of how to use a switch to method to handle window messages:
public IntPtr WndProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) { switch (msg) { case WM_PAINT: // Handle the WM_PAINT message. break; case WM_DESTROY: // Handle the WM_DESTROY message. break; default: // Handle any other messages. break; } return IntPtr.Zero;}
The `WndProc` method is the window procedure for the window. It is responsible for handling window messages. The `msg` parameter is the message that was sent to the window. The `wParam` and `lParam` parameters contain additional information about the message.
The `switch` statement in the `WndProc` method handles the different types of window messages. Each case in the `switch` statement handles a different type of message. The `default` case handles any messages that are not handled by the other cases.
The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully.
Return Type of Switch to Method in C# for Window Handles
The return type of a switch to method in C# for window handles is `IntPtr`. This is because window handles are represented as `IntPtr` values in C#.
- Representation: The return type of a switch to method in C# for window handles is `IntPtr` because window handles are represented as `IntPtr` values in C#.
- Window Procedure: The `WndProc` method is the window procedure for the window. It is responsible for handling window messages.
- Message Handling: The `switch` statement in the `WndProc` method handles the different types of window messages.
- Default Case: The `default` case in the `switch` statement handles any messages that are not handled by the other cases.
- Result: The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully.
- Example: Here is an example of how to use a switch to method to handle window messages:
public IntPtr WndProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) {switch (msg) {case WM_PAINT:// Handle the WM_PAINT message.break;case WM_DESTROY:// Handle the WM_DESTROY message.break;default:// Handle any other messages.break;}return IntPtr.Zero;}
These aspects highlight the importance of the return type of a switch to method in C# for window handles. By understanding the return type, developers can write more efficient and effective code to handle window messages.
Representation: The return type of a switch to method in C# for window handles is `IntPtr` because window handles are represented as `IntPtr` values in C#.
This statement establishes the fundamental connection between the return type of a switch to method in C# for window handles and the underlying representation of window handles as `IntPtr` values. It serves as the foundation for understanding the behavior and usage of switch to methods in this context.
- Data Type Compatibility
The `IntPtr` data type is used to represent window handles in C# because it can hold a pointer to an arbitrary memory location. This compatibility ensures that switch to methods can efficiently handle and manipulate window handles. - Native Interoperability
The use of `IntPtr` for window handles aligns with the native representation of window handles in the Windows API. This interoperability allows C# code to seamlessly interact with the underlying operating system and its window management mechanisms. - Performance Optimization
By using `IntPtr` as the return type, switch to methods can avoid unnecessary boxing and unboxing operations, which can improve performance, especially when dealing withwindow handles. - Type Safety
The `IntPtr` data type provides type safety by ensuring that window handles are handled correctly and cannot be inadvertently cast to other types, reducing the risk of errors and undefined behavior.
These facets collectively highlight the significance of the return type in switch to methods for window handles in C#. By understanding this connection, developers can leverage this mechanism effectively for window management and message handling tasks.
Window Procedure: The `WndProc` method is the window procedure for the window. It is responsible for handling window messages.
The `WndProc` method is the window procedure for the window. It is responsible for handling window messages. The return type of the `WndProc` method is `IntPtr`. This is because window handles are represented as `IntPtr` values in C#.
The `switch` statement in the `WndProc` method handles the different types of window messages. Each case in the `switch` statement handles a different type of message. The `default` case handles any messages that are not handled by the other cases.
The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully.
The following is an example of a `WndProc` method:
public IntPtr WndProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) { switch (msg) { case WM_PAINT: // Handle the WM_PAINT message. break; case WM_DESTROY: // Handle the WM_DESTROY message. break; default: // Handle any other messages. break; } return IntPtr.Zero;}
This `WndProc` method handles the `WM_PAINT` and `WM_DESTROY` messages. The `WM_PAINT` message is sent to the window when the window needs to be painted. The `WM_DESTROY` message is sent to the window when the window is being destroyed.
The `return` statement in the `WndProc` method returns `IntPtr.Zero` to indicate that the message was handled successfully.
Message Handling: The `switch` statement in the `WndProc` method handles the different types of window messages.
The `switch` statement in the `WndProc` method is crucial for handling window messages in C# for window handles. It allows the developer to specify different actions to be taken based on the type of message received by the window.
The return type of the `switch` statement is `IntPtr`, which is the representation of window handles in C#. This means that the result of handling a window message is a window handle.
The connection between the `switch` statement and the return type is important because it determines how window messages are processed and handled by the application. By understanding this connection, developers can write more efficient and effective code for window management and message handling.
For example, in a graphical user interface (GUI) application, the `WndProc` method can handle different types of window messages, such as mouse clicks, keyboard input, and window resizing. Each case in the `switch` statement can handle a specific type of message and perform the appropriate action, such as updating the window's appearance, processing user input, or resizing the window.
The return value of the `WndProc` method can be used to indicate the result of handling the message. For example, the method can return `IntPtr.Zero` to indicate that the message was handled successfully, or a non-zero value to indicate an error.
Overall, the connection between the `switch` statement and the return type of a switch to method in C# for window handles is essential for understanding how window messages are handled and processed in C# applications. By understanding this connection, developers can write more robust and efficient code for window management and message handling.
Default Case: The `default` case in the `switch` statement handles any messages that are not handled by the other cases.
The `default` case in the `switch` statement is essential for handling window messages in C# for window handles. It ensures that all window messages are processed, even if they are not explicitly handled by a specific case in the `switch` statement.
The return type of the `switch` statement is `IntPtr`, which is the representation of window handles in C#. This means that the result of handling a window message is a window handle.
When a window message is received by the window, the `WndProc` method is called to process the message. The `WndProc` method contains a `switch` statement that handles different types of window messages. Each case in the `switch` statement handles a specific type of message and performs the appropriate action, such as updating the window's appearance, processing user input, or resizing the window.
If the window message is not handled by any of the cases in the `switch` statement, the `default` case is executed. The `default` case can perform a default action, such as logging the message or passing it to another window procedure.
For example, in a graphical user interface (GUI) application, the `WndProc` method can handle different types of window messages, such as mouse clicks, keyboard input, and window resizing. Each case in the `switch` statement can handle a specific type of message and perform the appropriate action. The `default` case can handle any messages that are not handled by the other cases, such as custom messages or messages from other applications.
The `default` case is an important part of the `switch` statement for handling window messages in C# for window handles. It ensures that all window messages are processed, even if they are not explicitly handled by a specific case in the `switch` statement.
Understanding the connection between the `default` case and the return type of the `switch` statement is essential for writing efficient and effective code for window management and message handling.
Result: The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully.
The return type of a switch to method in C# for window handles is `IntPtr`. This is because window handles are represented as `IntPtr` values in C#. The `WndProc` method is the window procedure for the window. It is responsible for handling window messages. The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully.
- Message Handling
The `WndProc` method handles window messages. Each case in the `switch` statement handles a different type of message. The `default` case handles any messages that are not handled by the other cases. - Return Value
The `return` statement in the `WndProc` method returns the result of handling the message. The result is typically `IntPtr.Zero`, which indicates that the message was handled successfully. - Window Handle Representation
Window handles are represented as `IntPtr` values in C#. This is why the return type of a switch to method in C# for window handles is `IntPtr`. - Example
The following is an example of a `WndProc` method:public IntPtr WndProc(IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam) { switch (msg) { case WM_PAINT: // Handle the WM_PAINT message. break; case WM_DESTROY: // Handle the WM_DESTROY message. break; default: // Handle any other messages. break; } return IntPtr.Zero;}
These facets demonstrate the connection between the return type of a switch to method in C# for window handles and the result of handling the message. By understanding this connection, developers can write more efficient and effective code for window management and message handling.
Example: Here is an example of how to use a switch to method to handle window messages:
This example demonstrates the practical application of switch to methods for handling window messages in C#. By examining the code and its components, we can gain a deeper understanding of the return type of switch to methods in this context.
- Message Handling
This example showcases how switch to methods can handle various types of window messages. Each case in the switch statement is designed to handle a specific message type, such as WM_PAINT for repainting the window, or WM_DESTROY for handling window destruction. - Return Value
The return value of the switch to method is `IntPtr.Zero`, which signifies successful message handling. This return value is crucial for indicating the proper processing of window messages to the operating system. - Code Structure
The example illustrates the typical structure of a switch to method for window message handling. It includes the `switch` statement with message cases, `break` statements to exit each case, and a default case for handling unanticipated messages. - Event-Driven Programming
This example reflects the event-driven programming paradigm in Windows. Switch to methods enable the creation of message handlers that respond to specific window events, making the code more responsive and efficient.
By analyzing this example, we can appreciate the connection between the return type of switch to methods in C# for window handles and the practical implementation of window message handling. This example serves as a valuable reference for developers who wish to build robust and responsive window-based applications.
Frequently Asked Questions about Return Type of Switch to Method in C# for Window Handles
This section addresses common questions and misconceptions regarding the return type of switch to methods in C# for window handles.
Question 1: What is the return type of a switch to method for window handles in C#?
Answer: The return type of a switch to method for window handles in C# is `IntPtr`.
Question 2: Why is the return type `IntPtr`?
Answer: Window handles are represented as `IntPtr` values in C#, so the return type of a switch to method for window handles must also be `IntPtr`.
Question 3: What is the purpose of a switch to method for window handles?
Answer: A switch to method for window handles allows you to handle different types of window messages in a single method.
Question 4: How do I use a switch to method for window handles?
Answer: To use a switch to method for window handles, you must first create a `WndProc` method. The `WndProc` method is the window procedure for the window and is responsible for handling window messages. The `WndProc` method should contain a `switch` statement that handles the different types of window messages.
Question 5: What is the `default` case in a switch to method for window handles?
Answer: The `default` case in a switch to method for window handles handles any messages that are not handled by the other cases in the `switch` statement.
Question 6: What is the typical return value of a switch to method for window handles?
Answer: The typical return value of a switch to method for window handles is `IntPtr.Zero`. This indicates that the message was handled successfully.
These FAQs provide essential information about the return type of switch to methods for window handles in C#. Understanding these concepts is crucial for effectively handling window messages in C# applications.
Transitioning to the next article section: Understanding the nuances of switch to methods for window handles in C# enables developers to create robust and responsive window-based applications.
Conclusion
This article explored the return type of switch to method in C# for window handles. We learned that the return type is `IntPtr` because window handles are represented as `IntPtr` values in C#. We also examined the `WndProc` method, which is responsible for handling window messages, and the use of a `switch` statement to handle different types of window messages.
Understanding the return type of switch to method in C# for window handles is essential for writing efficient and effective code for window management and message handling. By leveraging this mechanism, developers can create robust and responsive window-based applications.
Leading WhatsApp For Nokia Lumia 8.1 - Get The App Today
Download GCC For Visual Studio: A Comprehensive Guide
Ultimate Guide To Denatured Alcohol Mixes With Ethanol
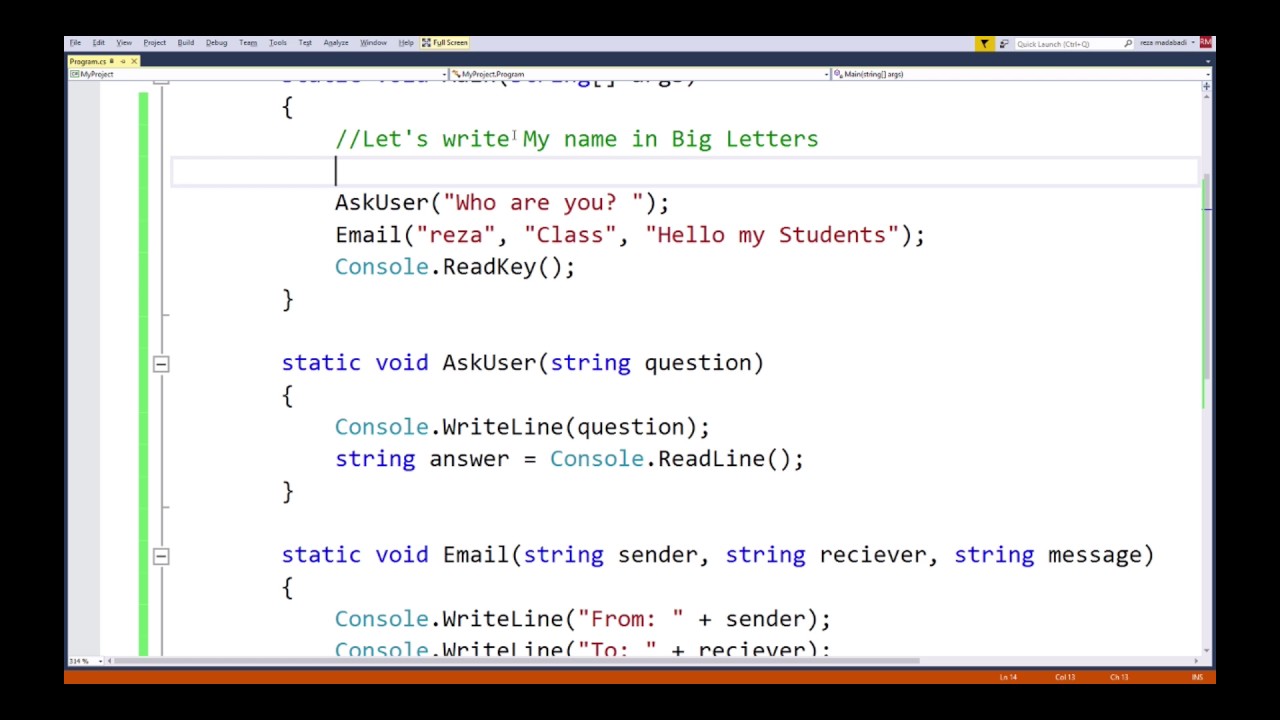
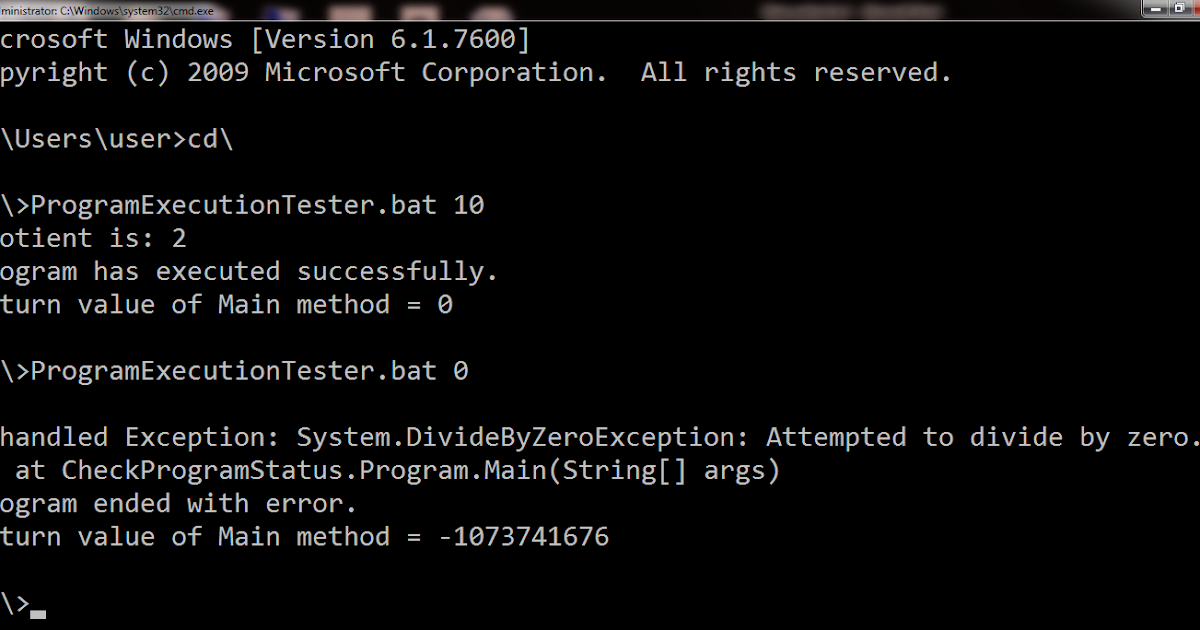